Microsoft Cognitive services is set of cloud based intelligence APIs for building richer and smarter application development. Cognitive API will use for Search meta data from Photos and video and emotions, sentiment analysis and authenticating speakers via voice verification.
The Computer Vision API will help developers to identify the objects with access to advanced algorithms for processing images and returning image meta data information. In this article, you will learn about Computer Vision API and how to implement Compute Vision API into Bot application.
You can follow below steps for implement object detection in Bot Application
Computer Vision API Key Creation
Computer Vision ApI returns information about visual content found in an image. You can follow below steps for create Vision API key.
Navigate to
https://azure.microsoft.com/en-us/try/cognitive-services/
Click on
“Get API Key “or Login with Azure login.
Login with Microsoft Account and Get API key
Copy API key and store securely, we will use this API key into our application
Step 2: Create New Bot Application
Let's create a new bot application using Visual Studio 2017. Open Visual Studio > Select File > Create New Project (Ctrl + Shift +N) > Select Bot application.
The Bot application template gets created with all the components and all required NuGet references installed in the solutions.
In this solution, we are going edit Messagecontroller and add Service class.
Install Microsoft.ProjectOxford.Vision Nuget Package
The Microsoft project oxford vision nuget package will help for access cognitive service so Install “
Microsoft.ProjectOxford.Vision” Library from the solution
Create Vision Service: Create new helper class to the project called VisionService that wraps around the functionality from the VisionServiceClient from Cognitive Services and only returns what we currently need.
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Threading.Tasks;
using System.Web;
using Microsoft.ProjectOxford.Vision;
using Microsoft.ProjectOxford.Vision.Contract;
namespace BotObjectDetection.Service
{
public class VisionService : ICaptionService
{
/// <summary>
/// Microsoft Computer Vision API key.
/// </summary>
private static readonly string ApiKey = "<API Key>";
/// <summary>
/// The set of visual features we want from the Vision API.
/// </summary>
private static readonly VisualFeature[] VisualFeatures = { VisualFeature.Description };
public async Task<string> GetCaptionAsync(string url)
{
var client = new VisionServiceClient(ApiKey);
var result = await client.AnalyzeImageAsync(url, VisualFeatures);
return ProcessAnalysisResult(result);
}
public async Task<string> GetCaptionAsync(Stream stream)
{
var client = new VisionServiceClient(ApiKey);
var result = await client.AnalyzeImageAsync(stream, VisualFeatures);
return ProcessAnalysisResult(result);
}
/// <summary>
/// Processes the analysis result.
/// </summary>
/// <param name="result">The result.</param>
/// <returns>The caption if found, error message otherwise.</returns>
private static string ProcessAnalysisResult(AnalysisResult result)
{
string message = result?.Description?.Captions.FirstOrDefault()?.Text;
return string.IsNullOrEmpty(message) ?
"Couldn't find a caption for this one" :
"I think it's " + message;
}
}
} In the above helper class, replace vision API key and call the Analyze image client method for identify image meta data
Messages Controller
MessagesController is created by default and it is the main entry point of the application. it will call our helper service class which will handle the interaction with the Microsoft APIs. You can update “Post” method like below
using System;
using System.Diagnostics;
using System.IO;
using System.Linq;
using System.Net;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Text.RegularExpressions;
using System.Threading.Tasks;
using System.Web.Http;
using BotObjectDetection.Service;
using Microsoft.Bot.Builder.Dialogs;
using Microsoft.Bot.Connector;
namespace BotObjectDetection
{
[BotAuthentication]
public class MessagesController : ApiController
{
private readonly ICaptionService captionService = new VisionService();
/// <summary>
/// POST: api/Messages
/// Receive a message from a user and reply to it
/// </summary>
public async Task<HttpResponseMessage> Post([FromBody]Activity activity)
{
if (activity.Type == ActivityTypes.Message)
{
//await Conversation.SendAsync(activity, () => new Dialogs.RootDialog());
var connector = new ConnectorClient(new Uri(activity.ServiceUrl));
string message;
try
{
message = await this.GetCaptionAsync(activity, connector);
}
catch (Exception)
{
message = "I am object Detection Bot , You can Upload or share Image Url ";
}
Activity reply = activity.CreateReply(message);
await connector.Conversations.ReplyToActivityAsync(reply);
}
else
{
HandleSystemMessage(activity);
}
var response = Request.CreateResponse(HttpStatusCode.OK);
return response;
}
private Activity HandleSystemMessage(Activity message)
{
if (message.Type == ActivityTypes.DeleteUserData)
{
// Implement user deletion here
// If we handle user deletion, return a real message
}
else if (message.Type == ActivityTypes.ConversationUpdate)
{
// Handle conversation state changes, like members being added and removed
// Use Activity.MembersAdded and Activity.MembersRemoved and Activity.Action for info
// Not available in all channels
}
else if (message.Type == ActivityTypes.ContactRelationUpdate)
{
// Handle add/remove from contact lists
// Activity.From + Activity.Action represent what happened
}
else if (message.Type == ActivityTypes.Typing)
{
// Handle knowing tha the user is typing
}
else if (message.Type == ActivityTypes.Ping)
{
}
return null;
}
private async Task<string> GetCaptionAsync(Activity activity, ConnectorClient connector)
{
var imageAttachment = activity.Attachments?.FirstOrDefault(a => a.ContentType.Contains("image"));
if (imageAttachment != null)
{
using (var stream = await GetImageStream(connector, imageAttachment))
{
return await this.captionService.GetCaptionAsync(stream);
}
}
string url;
if (TryParseAnchorTag(activity.Text, out url))
{
return await this.captionService.GetCaptionAsync(url);
}
if (Uri.IsWellFormedUriString(activity.Text, UriKind.Absolute))
{
return await this.captionService.GetCaptionAsync(activity.Text);
}
// If we reach here then the activity is neither an image attachment nor an image URL.
throw new ArgumentException("The activity doesn't contain a valid image attachment or an image URL.");
}
private static async Task<Stream> GetImageStream(ConnectorClient connector, Attachment imageAttachment)
{
using (var httpClient = new HttpClient())
{
var uri = new Uri(imageAttachment.ContentUrl);
return await httpClient.GetStreamAsync(uri);
}
}
private static bool TryParseAnchorTag(string text, out string url)
{
var regex = new Regex("^<a href=\"(?<href>[^\"]*)\">[^<]*</a>$", RegexOptions.IgnoreCase);
url = regex.Matches(text).OfType<Match>().Select(m => m.Groups["href"].Value).FirstOrDefault();
return url != null;
}
}
}
Run Bot Application
The emulator is a desktop application that lets us test and debug our bot on localhost. Now, you can click on "Run the application" in Visual studio and execute in the browser
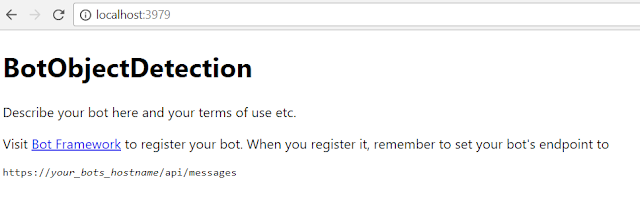
Test Application on Bot Emulator
You can follow the below steps to test your bot application.
Open Bot Emulator.
Copy the above localhost url and paste it in emulator e.g. -
http://localHost:3979 You can append the /api/messages in the above url; e.g. -
http://localHost:3979/api/messages. You won't need to specify Microsoft App ID and Microsoft App Password for localhost testing, so click on "
Connect".
Related Article: I have explained about Bot framework Installation, deployment and implementation in the below article
Summary
In this article, you learned how to create an Intelligent Image Object Detection Bot using Microsoft Cognitive Computer Vision API. If you have any questions/feedback/ issues, please write in the comment box.
Enable GingerCannot connect to Ginger Check your internet connection
or reload the browserDisable in this text fieldEditEdit in GingerEdit in Ginger
The Bot Application runs in an application, like Skype, web chat, Facebook, Message, etc. Users can interact with bots by sending them messages, commands, and inline requests. You control your bots using HTTPS requests to the bot API.
The better visibility, the higher will be the chance of customers making a purchase. Unlike other bots which are available on business web pages which provide visibility only to the customers visiting their site, a Facebook bot is very easy to find. It is visible to around 1.3 billion users of Facebook. A Facebook bot interacts very well with customers. It can receive and send messages, images and provide clickable buttons like Buy Now. In this article, I am going to show how we can connect Facebook Messenger's channel and integrate Bot Application to the Messenger app.
In this Article, I am going to show how we can connect Facebook messengers’ channel and integrate Bot Application to the messengers App.
Create FAQ Bot Application
You can refer
my previews article to create and build a Xamarin FAQ Bot using the Azure Bot Service and deploy it into Azure. I am not using any coding for developing the Bot Application, you can follow the steps provided in the article to create and deploy FAQ Bot.
Setup Facebook Page
We can implement Bot Application to the Facebook page. You can
create Facebook page or select existing page and navigate to “About page” for fining and copy the Page ID.
Login to Facebook APP
Create a new Facebook App (
https://bit.ly/1D0BHpg) on the Page and generate an App ID
, App Secret and Page Access Token for integrating Bot to the page messenger. You can click on “Skip and Create APP Id” from following screen.
Create New App ID
Provide display Name and Contact Email for integrating Bot application and Click on Create.
After Create button, it will navigate to the App dashboard screen. The side navigation menu having Settings > Basic and copy the APPID and APP Secret. Provide the Privacy URL, Terms of Service URL, App icon and Select Category.
I have shown the following screen to Select Setting > Advanced and Set the "Allow API Access to App Settings slider to "Yes" and click on Save Changes.
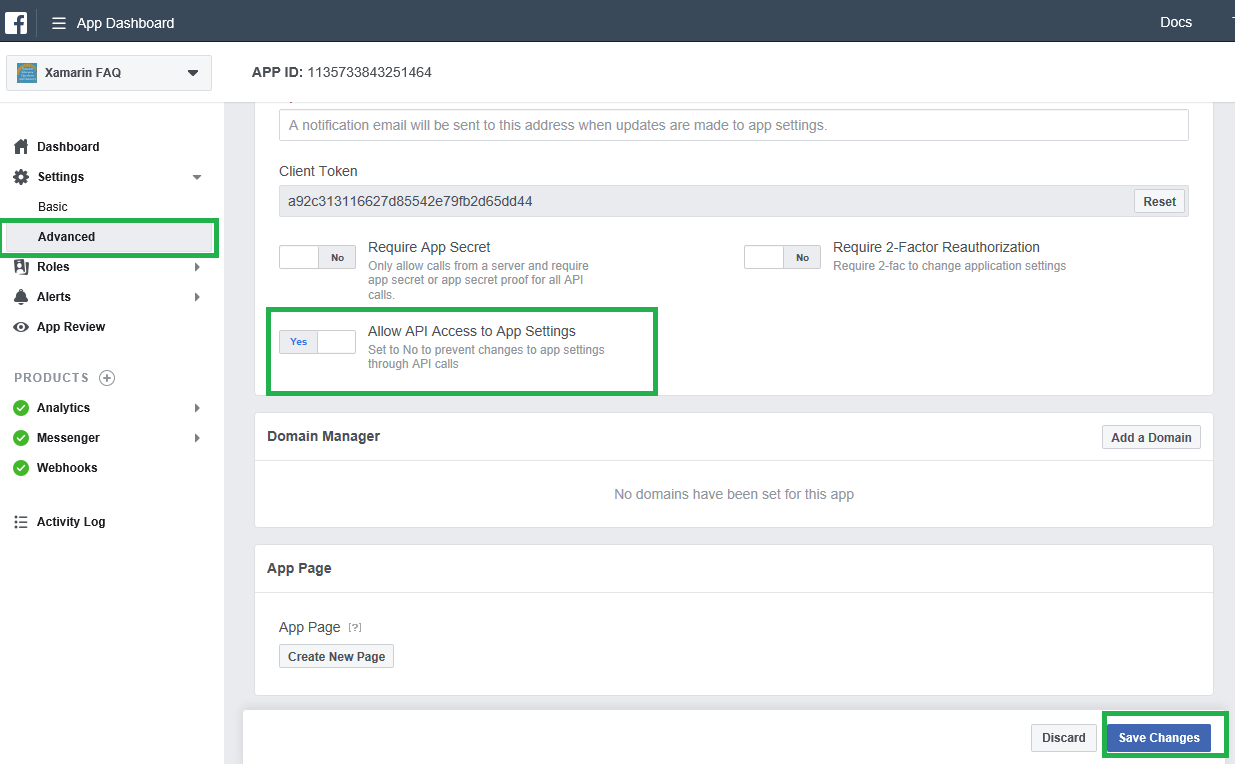
Setup Message
Select Dashboard and Click on Setup button from messenger group
Create Page Access Token
Select Setting from the messenger side navigation menu to generate token, you can select the Page and generate access token and copy the page access token.
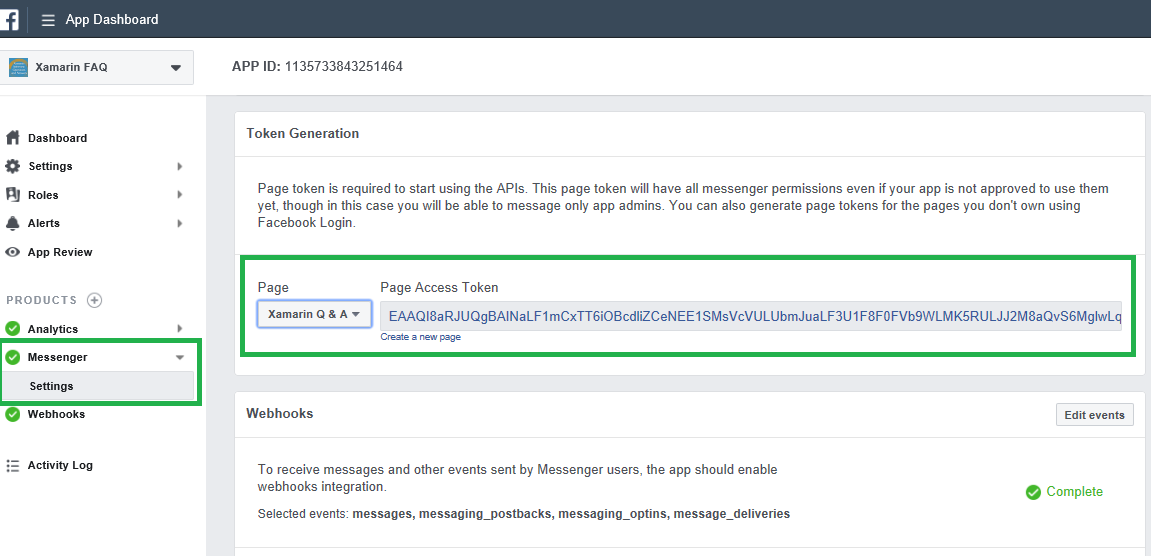
Setup Webhooks
Click Set up Webhooks to forward messaging events from Facebook Messenger to the bot.
Provide following callback URL and Verify
token to the
webhooks setup page and select the message, message_postbacks, messaging_optins and message_deliveries subscription fields. The following Steps 2, will show how to generate Callback URL and verify
token from Azure portal.
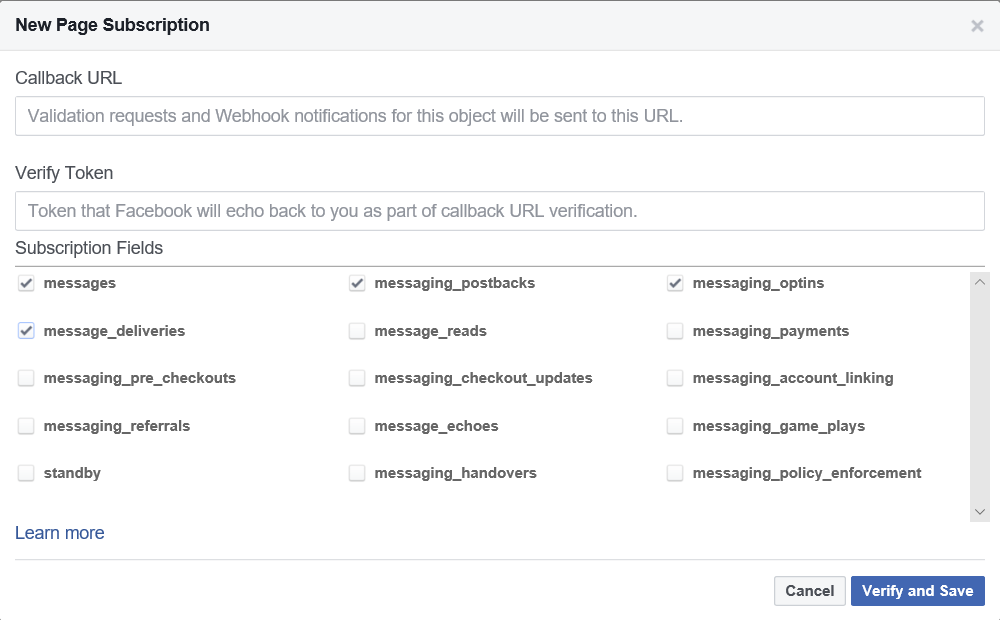
You can click on “Verify and Save “and select the Facebook page to Subscribe the
webhook to the Facebook page.
Connect Facebook Channel
Step 1: Login to Azure portal > Select the “All Resources” > Select Channels > Select Facebook Messengers, let
we start configure “Facebook Messengers “Channel and follow below steps, the end of this article you can able to deploy Bot into the Facebook messenger
Step 2:The
Azure Facebook configuration channel will generate following Callback URL and verify token, You can copy those information and Past to the Facebook
webhook setup screen.
(Return to the Facebook messenger setup screen).
Step 3: You can paste the Facebook App ID, Facebook App Secret, Page ID
,and Page Access Token values copied from Facebook Messenger previously. You can use the same bot on multiple
facebook pages by adding additional page ids and access tokens.
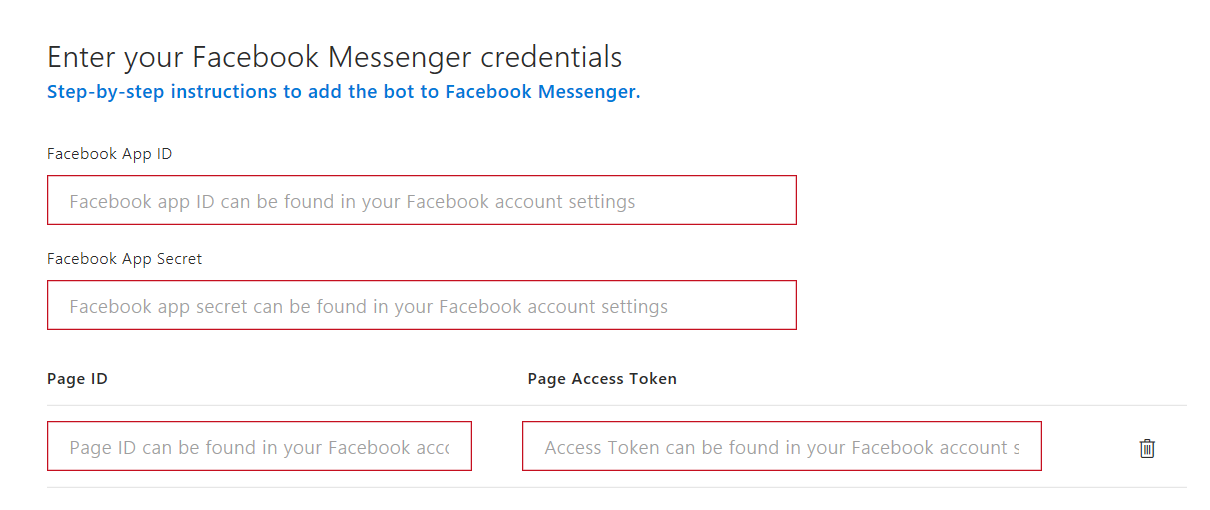
Submit to Facebook Review
Select App preview and Click and Submit for review after submit will take some time for the
facebook team testing to the messenger bot and you can mark your app live available to the public
, then you can test messenger from the Facebook page
.
Facebook Team reviews and Testing
You can verify Facebook team testing progress, navigate to your Facebook page and Click on Inbox and verify, if anything problem
to the messenger bot, the Team will update the bug list from App review screen.
Xamarin FAQ Messengers testing
You can select your Facebook page and test your bot application. I have trained 7000+ more questions to the Facebook messenger bot from Xamarin Q & A Page, If you want to look the
xamarin FAQ demo. Navigate to Xamarin Q & A Facebook page and ask your
xamairn related question.
Summary
In this article, you have learned how to integrate bot application right into your Facebook Page via Azure Microsoft AI. If you have any questions/feedback/issues, please write in the comment box.
Microsoft Cognitive Services offers awesome APIs and services for developers to create more intelligent applications. You can add interesting features, like people's emotions and video detection, face, speech, and vision recognition and speech and language understanding into your application.
The Emotion API takes a facial expression in an image stream as an input and returns confidence levels across a set of emotions for each face in the image like anger, contempt, disgust, fear, happiness, neutral, sadness, and surprise, in a facial expression
Where to implement
There is no single unifying definition or theory of emotion. Nevertheless, several characteristics of an emotional reaction are frequently mentioned. Nowadays feedback is more important for product promotion and review the article, store, etc. manual entry of emotion maybe wrong so we can automate the feedback and review.
In this blog, we can learn about how to implement Emotion Recognition in Xamarin.Forms with Microsoft Cognitive Services.
Getting Started with the Emotion API
The Emotion API can detect anger, contempt, disgust, fear, happiness, neutral, sadness, and surprise, in a facial expression. As well as returning an emotion result for a facial expression, the Emotion API also returns a bounding box for detected faces using the Face API. If a user has already called the Face API, they can submit the face rectangle as an optional input. Note that an API key must be obtained to use the Emotion API. You can follow below steps for obtained at free API key on Microsoft.com
Step 1: Click
here for Generate Subscriber Key and Click on Create
Step 2: You can agree the terms and Microsoft privacy statement > select the country and click on Next
Step 3: Login with Microsoft /LinkedIn /Facebook /Github for create your API
Step 4: You login and subscribe you should see the keys and the below information on available your subscriptions
We will use emotion API key in the following Xamarin application. before that we can test the API key
Test on Your Emotion Key
You can navigate emotion API
online console application and provide the subscription key and image url . If your provide valid key and input the image, you will get it JSon data as output or relevant error
Create New Xamarin Forms Application
You can refer
my previous article for setup and create new Xamarin forms application.
If you already installed VS on your machine, Open Visual Studio > Create New Xamarin Forms application like below
Solution will create with all the platform and PCL project
In this solution, we are going to create demo application for Article review automate based on the emotion.
Camera in Xamarin. Forms
We can use Media.Plugin Nuget package for Capture image or select image gallery from all the mobile platform (iOS,Android,Windows)
Right Click on Solution > Type “xam.plugin” in the search box > Select all the project (PCL, iOS, Android and UWP) > Click on Install
Emotion Nuget Package
This client library is a thin C# client wrapper for the Microsoft Emotion API.The easiest way to use this client library is to get microsoft.projectoxford.emotion package from nuget .
Right Click on Solution > Type “Microsoft Emotion” in the search box > Select all the project (PCL, iOS, Android and UWP) > Click on Install
UI Design
After successfully install above two nuget package. Let start UI design from PCL project In Dotnet Standard Project > Open MainPage.Xaml page and design following xaml code
<?xml version="1.0" encoding="utf-8" ?>
<ContentPage xmlns="http://xamarin.com/schemas/2014/forms"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
xmlns:local="clr-namespace:DevEnvExeEmotion"
x:Class="DevEnvExeEmotion.MainPage">
<StackLayout Margin="20">
<StackLayout Orientation="Horizontal" Padding="30" HorizontalOptions="CenterAndExpand" >
<StackLayout x:Name="emotion">
<Label Text="Reader is :" FontSize="Large"/>
<Label x:Name="emotionResultLabel" FontSize="Large" />
</StackLayout>
<Button Text="Share Your FeedBack" Clicked="OnFeedBackClicked"></Button>
</StackLayout>
<Image x:Name="image" Source="article.png" />
<ActivityIndicator x:Name="activityIndicator" />
</StackLayout>
</ContentPage>
In mainPage.xaml.cs file add your logic.
public partial class MainPage : ContentPage
{
EmotionServiceClient emotionClient;
MediaFile photo;
public MainPage()
{
InitializeComponent();
emotion.IsVisible = false;
emotionClient = new EmotionServiceClient("7f495be5faf643adbeead444d5b79a13");
}
For capture image, write the following code
public async Task CaptureImage()
{
await CrossMedia.Current.Initialize();
emotion.IsVisible = false;
// Take photo
if (CrossMedia.Current.IsCameraAvailable || CrossMedia.Current.IsTakePhotoSupported)
{
photo = await CrossMedia.Current.TakePhotoAsync(new StoreCameraMediaOptions
{
SaveToAlbum = false
});
}
else
{
await DisplayAlert("No Camera", "Camera unavailable.", "OK");
}
}
For Recognize emotion, write the following code
public async Task Recognizeemotion()
{
try
{
if (photo != null)
{
using (var photoStream = photo.GetStream())
{
Emotion[] emotionResult = await emotionClient.RecognizeAsync(photoStream);
if (emotionResult.Any())
{
// Emotions detected are happiness, sadness, surprise, anger, fear, contempt, disgust, or neutral.
emotionResultLabel.Text = emotionResult.FirstOrDefault().Scores.ToRankedList().FirstOrDefault().Key;
emotion.IsVisible = true;
}
photo.Dispose();
}
}
}
catch (Exception ex)
{
Debug.WriteLine(ex.Message);
}
}
While click on Feedback button, call the above two methods
async void OnFeedBackClicked(object sender, EventArgs e)
{
//capture image
await CaptureImage();
((Button)sender).IsEnabled = false;
// Recognize emotion
await Recognizeemotion();
((Button)sender).IsEnabled = true;
}
Run the Application
Select the iOS /Android /Windows and install into the device and click on ‘Share your Feedback ‘button
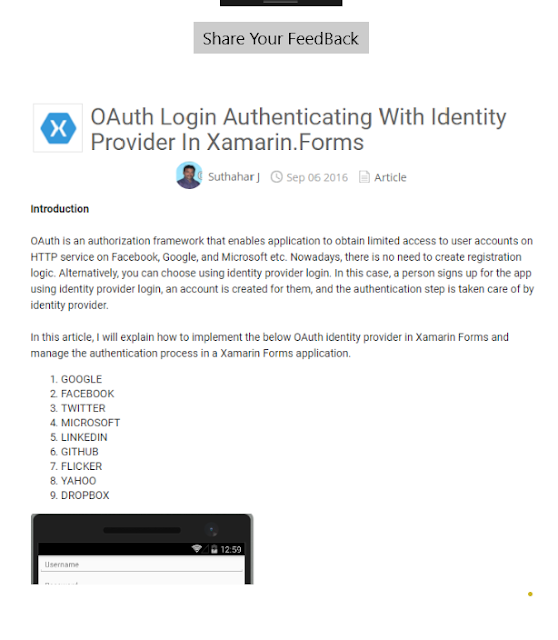
The device should be camera so after click on the button, capture your face for Recognize emotion.
Summary
In this article, you learned about how to generate emotion API key and implement Emotion Recognition in Xamarin.Forms with Microsoft Cognitive Services.If you have any questions/ feedback/ issues, please write in the comment box.
Enable GingerCannot connect to Ginger Check your internet connection
or reload the browserDisable in this text fieldEditEdit in GingerEdit in Ginger
Sentiment analysis seeks to understand a subject’s attitude or emotional reaction towards a specific topic or brand. Sentiment analysis does not have to be complicated and technical. It could be something as simple as getting a person in your team to find what is being said about your brand and product on review page and identify how much of it is good and how much of isn’t. There is no need for a big budget and a developer into complicated software, the cognitive service text Analytics API is a cloud-based service that provides advanced natural language processing over raw text, and includes four main functions: sentiment analysis, key phrase extraction, language detection, and entity linking.
The most of the companies and brands now use sentiment analysis to find out what people are saying about them on social media. A bad review of social media can destroy a brand’s reputation if ignored or poorly handled. They aren’t simply rating their experience with 1 star or 5 stars. They’re also expressing their thoughts, feeling, expectations in free form text. This can be challenging to handle, especially if your company is getting a lot of feedback. When you have tens or even hundreds of thousands of feedbacks to read and manage, its easy to use cognitive text analytics service API.
The Sentiment Analysis API evaluates text input and returns a sentiment score for each document, ranging from 0 (negative) to 1 (positive). This capability is useful for detecting positive and negative sentiment in social media, customer reviews, and discussion forums. Content is provided by you, models and training data are provided by the service.
Currently, Sentiment Analysis supports English, German, Spanish, and French. Other languages are in the preview. In this article, I will show how we can integrate sentiment API from Xamarin Mobile application using visual studio 2019
Text Analytics API Price
The Text Analytics API can be purchased in units of the S0-S4 tier at a fixed price. Each unit of a tier comes with included quantities of API transactions. If the user exceeds the included quantities, overages are charged at the rate specified in the pricing table below. These
overages are prorated and the service is billed on a monthly basis. The included quantities in a tier are reset each month. In the S tier, the service is billed for only the amount of Text Records submitted to the service. You can read more about pricing based on the country check out here
.
Create Text Analytics API Key
You need to create an Azure account, generate API key and end point URL based region for implementation to the Xamarin Mobile application.
Step 2: Create On “+ Create a resource “> Under Azure Marketplace, select AI + Machine learning and discover the list of available featured. > Select “Text Analytics”
Step 3: On the create page, provide the name, pricing, resource group and click on Create
Step 4:Wait for a few seconds. After the Cognitive Services account is successfully deployed, click the notification or tile in the dashboard to view the account information. You can copy the Endpoint URL and Key in the Overview section for API calls in our Xamarin applications.
Build Xamarin Forms Application using Visual Studio 2019
Let's start with creating a new Xamarin Forms Project using Visual Studio 2019. When accessing Visual Studio 2019 for the first time, you will come across a new interface for opening a creating the project.
Open Run >> Type “Devenev
.Exe” and enter >> Create New Project (Ctrl+Shift+N) or select open recent application.
The available templates will appear
on a window like below. Select Xamarin Forms application with different mobile platform.
Provide project name, Location and solution name in the following configure new project screen
Select as Blank apps and select the platform
The Solution will be created with all the platform and PCL projects.
Xamarin UI Design:
The UI will have a few elements on the screen and overlay content view window. Editor control for providing user input value and an overlay window to show the result.
You can add Newtonsoft.JSON to solutions. Right click on Solutions > Manage NuGet Packages > select Newtonsoft.Json from Browse tab > click on Install.
<?xml version="1.0" encoding="utf-8" ?>
<ContentPage xmlns="http://xamarin.com/schemas/2014/forms"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
xmlns:local="clr-namespace:MobileFeedback"
:Class="MobileFeedback.MainPage">
<ContentPage.Content>
<AbsoluteLayout>
<!-- Normal Page Content -->
<StackLayout AbsoluteLayout.LayoutBounds="0, 0, 1, 1"
AbsoluteLayout.LayoutFlags="All">
<Image Source="product.gif" VerticalOptions="Start" HorizontalOptions="Start" Margin="0,0,0,0" ></Image>
<Editor x:Name="txtfeedback" WidthRequest="100" HeightRequest="200"></Editor>
<Button Text="Submit" Clicked="Submit_Clicked"></Button>
</StackLayout>
<!-- Overlay -->
<ContentView x:Name="overlay"
AbsoluteLayout.LayoutBounds="0, 0, 1, 1"
AbsoluteLayout.LayoutFlags="All"
IsVisible="False"
BackgroundColor="#C0808080"
Padding="10, 0">
<StackLayout Orientation="Vertical"
BackgroundColor="White"
HeightRequest="175"
WidthRequest="300"
HorizontalOptions="Center"
VerticalOptions="Start"
Margin="0,20,0,0" >
<Image x:Name="imgstatus" WidthRequest="70" HeightRequest="70"></Image>
<Label Text="" x:Name="lblStatus"></Label>
<StackLayout Orientation="Horizontal" HorizontalOptions="Center">
<Button Text="OK" FontSize="Small"
VerticalOptions="CenterAndExpand"
HorizontalOptions="Center"
Clicked="OnOKButtonClicked" />
</StackLayout>
</StackLayout>
</ContentView>
</AbsoluteLayout>
</ContentPage.Content>
</ContentPage>
Create Document Entity Class
Create a class for Document class, it will deserialize the response and return an object of type TextAnalyticsResponse.The response format defined by the API looks like below document entity
using System;
using System.Collections.Generic;
using System.Text;
namespace MobileFeedback
{
class Document
{
public string Id { get; set; }
public double? Score { get; set; }
}
class TextAnalyticsResponse
{
public List<Document> Documents { get; set; }
}
}
Create SentimentAnalysisHelper Helper Class
You can replace Text Analytics API service endpoint and subscription key. If you don't already have these go back to the previous steps. Below is the complete class you need to add. We have to append /sentiment to the end of the ApiUri in order to invoke the sentiment operation.
using Newtonsoft
.Json;
using System;
using System
.Collections
.Generic;
using System
.Net
.Http;
using System
.Text;
namespace MobileFeedback
{
static class SentimentAnalysisHelper
{
private const string ApiUri = "<API url>”;
private const string
SubscriptionKey = "<your Key>";
private const string Text = "The food was delicious and there were wonderful staff.";
private static
readonly HttpClient Client = GetClient
();
private static HttpClient GetClient
()
{
var client = new HttpClient
();
client.DefaultRequestHeaders
.Add
("Ocp-Apim-Subscription-Key",
SubscriptionKey);
client.DefaultRequestHeaders
.Add
("ContentType", "application/json");
client.DefaultRequestHeaders
.Add
("Accept", "application/json");
return client;
}
private static TextAnalyticsResponse DeserializeTextAnalyticsResponse
(string
json)
{
return JsonConvert
.DeserializeObject<TextAnalyticsResponse>(
json);
}
public static TextAnalyticsResponse GetSentiment
(string text)
{
var body = JsonConvert
.SerializeObject
(new
{
Documents = new object
[]
{
new
{
Text = text,
Id =
Guid.NewGuid
()
}
}
});
using (
var content = new ByteArrayContent
(Encoding.UTF8.GetBytes
(body)))
{
var responseMessage = Client.PostAsync(ApiUri, content).Result;
responseMessage.EnsureSuccessStatusCode
();
var json =
responseMessage.Content
.ReadAsStringAsync
()
.Result;
return DeserializeTextAnalyticsResponse
(json);
}
}
}
}
You can add following to code behind in the design file.
we'll add a method
(GetSentiment) to call the Text Analytics API sentiment endpoint. It will
deserialize the response and return an object of type TextAnalyticsResponse
.The method will take a string text as input, create the request body, and then send it to the Text Analytics API using an HttpClient instance.
using System;
using System
.Collections
.Generic;
using System
.Linq;
using System
.Text;
using System
.Threading
.Tasks;
using Xamarin
.Forms;
using System
.Net
.Http;
using System
.Threading;
namespace MobileFeedback
{
public partial class MainPage
: ContentPage
{
public MainPage
()
{
InitializeComponent
();
}
private void Submit_Clicked
(object sender, EventArgs e)
{
TextAnalyticsResponse result = SentimentAnalysisHelper
.GetSentiment
(txtfeedback.Text);
double?
score = result
.Documents
.FirstOrDefault
()
.Score;
if(score < 0.5)
{
imgstatus.Source = "bad.png";
lblStatus.Text = "This is the first time we have heard of this problem. Thank you for pointing it out to us. I assure you we will do our best to prevent it from happening again, We will contact with you
regardng the issue";
}
else if
(score > 0.5 && score < 0.9)
{
imgstatus.Source = "okay.png";
lblStatus.Text = "We are always eager to get feedback from our customers. Thank you for taking the time to write to us
.We will improve our service and our team will contact you";
}
else if
(score> 0.9)
{
imgstatus.Source = "happy.png";
lblStatus.Text = "Your valuable feedback will assist us in our continuing effort to provide our users with the best possible support experience.";
}
overlay.IsVisible = true;
}
void OnOKButtonClicked
(object sender, EventArgs args)
{
overlay.IsVisible = false;
}
void OnCancelButtonClicked
(object sender, EventArgs args)
{
overlay.IsVisible = false;
}
}
}
We have completed the code for consuming TextcAnalytics API. Now, we can select the platform and press F5. The output looks like below
Summary
In this article, you learned how to consume Text Analytics API and automate customer feedback without using rating. I hope this article will help you. Please leave your feedback/query using the comments box, if you like this article, please share it with your friends.