Improving C# Performance by Using AsSpan and Avoiding Substring
During development and everyday use, Substring is often the go-to choice for string manipulation. However, there are cases where Substring can negatively impact performance and result in unnecessary string memory allocation.
In this article, we will explore how to enhance the performance of C# applications by making informed decisions when working with substrings.
We'll compare the traditional Substring method with the newer AsSpan method and discuss when to use each for maximum efficiency
Substring()
The Substring method is a commonly used tool for extracting substrings from a string in C#. While it's convenient and easy to use.I have created a sample method that takes a full date string, splits the string, and returns the day, month, and year separated by commas.
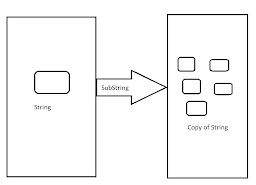
This can result in additional memory allocations, which can be problematic, particularly when handling large strings or frequent substring operations.
Same date full string convert into AsSpan method
🐌 Memory Allocation
When you call Substring, it creates a new string object and copies the characters from the original string to the new substring.This can result in additional memory allocations, which can be problematic, particularly when handling large strings or frequent substring operations.
AsSpan
In contrast to Substring, the AsSpan method returns a ReadOnlySpan from the original string. A ReadOnlySpan is a lightweight, stack-allocated view over the data, and it doesn't create a new string object or copy the characters.Same date full string convert into AsSpan method
🚀 Improved Performance:
By using AsSpan, you can significantly improve the performance of your code. It eliminates the overhead associated with creating new string objects and copying characters, making it an excellent choice for scenarios where performance is critical.
Reduced Memory Overhead: The lack of memory copying in AsSpan means that your application consumes less memory, reducing the load on the garbage collector and leading to a more efficient use of resources. You can refer to the statistics below for the results of the two methods.

Reduced Memory Overhead: The lack of memory copying in AsSpan means that your application consumes less memory, reducing the load on the garbage collector and leading to a more efficient use of resources. You can refer to the statistics below for the results of the two methods.
When I can use AsSpan
The AsSpan method is particularly valuable in situations where you need to work with portions of a string without the need for actual string manipulation. Here are some common scenarios where using AsSpan is recommended💡 API Compatibility:
Many APIs that accept strings now have overloads that accept a ReadOnlySpan argument. When such overloads are available, you can improve performance by calling AsSpan instead of Substring.
Performance-Critical Operations:
If your application performs extensive string manipulation operations, consider using AsSpan for better performance.
When I can use Substring
It's essential to note that there are still situations where using Substring may be appropriate✅ Creating a Copy
If you need to create a distinct copy of the substring (rather than a view), Substring remains a suitable choice.
0 Comments