Getting started with Microsoft Graph API in a Xamarin Forms Application
Introduction:
Microsoft offer a different service in the Cloud, Mail, Calendar, Contact, Chat and files from common Microsoft portal and also if you want to integrate to your application, you can access unified API wrapper in the Microsoft graph SDK. In this article you will get understand, how to implement login authentication and Send mail using Microsoft graph API in the Xamarin Forms applicationPrerequisites:
Download and install Visual 2017 Community Edition or higher.Create Microsoft Account (If you already register Microsoft account, it’s not required)
Getting Start Create Application:
Step 1:
You can navigate following URL https://developer.microsoft.com/en-us/graph/quick-start for register app and create sample app.
Step 2:
Select the platform and Microsoft will create simple app that connects to Office 365 and calls the Microsoft Graph API.

Step 3:
Register APP ID: You can register the application using click on “Get an app ID” and it will navigate to Microsoft application registration portal where you will be able to get an App ID and redirect URL. You will need either a School / Work /corporate / Azure AD or Microsoft account.

Step 4:
Registration Success: after login with your Microsoft account and wait for few second appid and redirect url will generate automatically. if you want to modify or manage your app, you can click on application registration portal .

Step 5:
Manage App in Registration Portal: You can manage or edit application from portal in the following App Name, redirect URL, generate new password, application logo, update the permission and click on Save button.
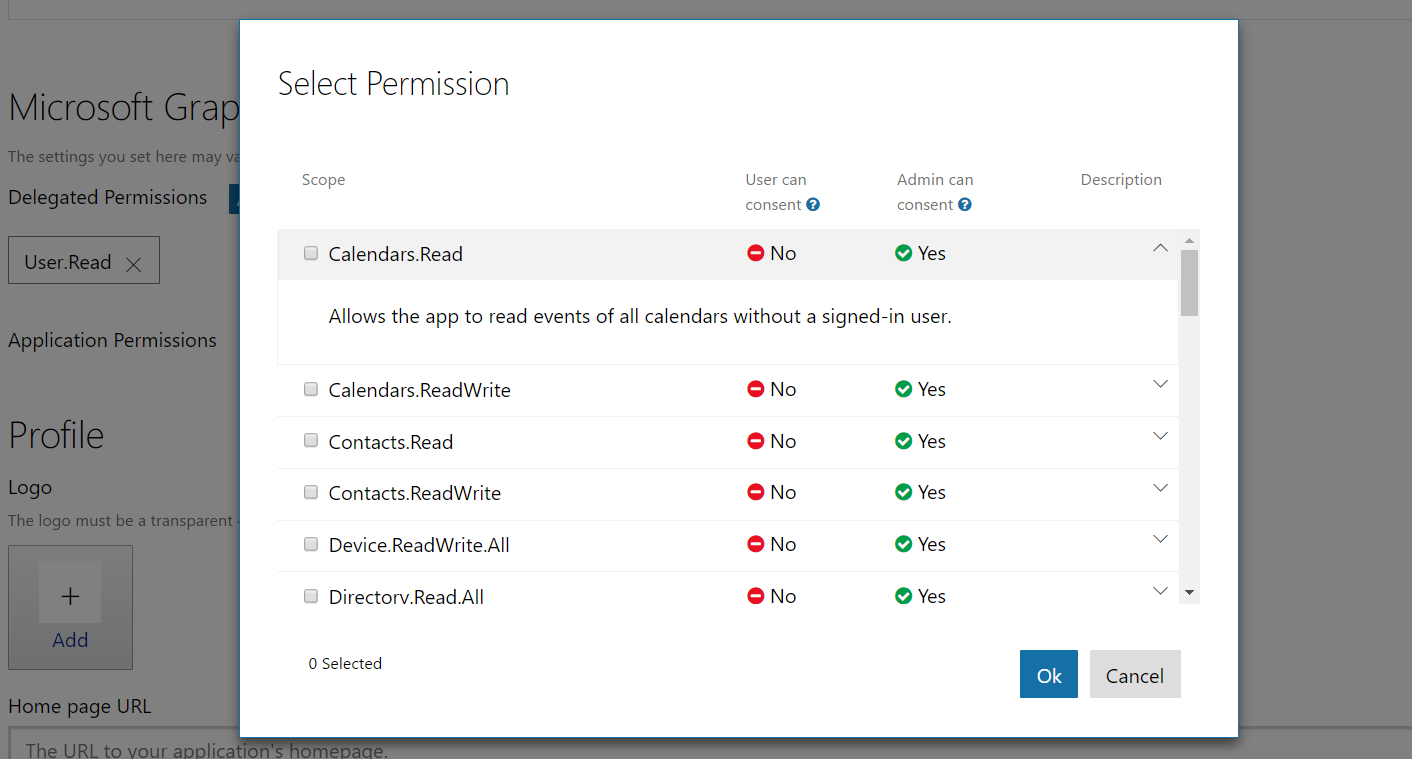
Step 6:
Download Sample Code: you can download and unzip the source code > open the source code using Visual Studio. in the Sample application have multiple platform support as iOS, Android, UWP. The code sample will introduce you to authentication and send an email from your account.
Step 7:
Microsoft Graph Client Library:
Microsoft Graph Client Library allows you to call Office 365, Azure AD and other Microsoft services through a single unified developer experience so right click on your solution and verify below NuGet package installed in the solution.Step 8:
Configure the project: Open the App.cs file from XamarinConnect PCL project > replace your client ID and add the user permission scope.
public static string ClientID = "< Update your Client ID > ";
public static PublicClientApplication IdentityClientApp = new PublicClientApplication(ClientID);
public static string[] Scopes = { "User.Read", "Mail.Send", "Files.ReadWrite" };
public static PublicClientApplication IdentityClientApp = new PublicClientApplication(ClientID);
public static string[] Scopes = { "User.Read", "Mail.Send", "Files.ReadWrite" };
Step 9:
Configure Send mail: Open the MainHelper.cs from XamarinConnect PCL project > update ComposeAndSendMailAsync method as per your requirement
public async Task ComposeAndSendMailAsync(string subject,string bodyContent,string recipients)
{
// Get current user photo
Stream photoStream = await GetCurrentUserPhotoStreamAsync();
// If the user doesn't have a photo, or if the user account is MSA, we use a default photo
if (photoStream == null)
{
var assembly = typeof(MailHelper).GetTypeInfo().Assembly;
photoStream = assembly.GetManifestResourceStream("XamarinConnect.test.jpg");
}
MemoryStream photoStreamMS = new MemoryStream();
// Copy stream to MemoryStream object so that it can be converted to byte array.
photoStream.CopyTo(photoStreamMS);
DriveItem photoFile = await UploadFileToOneDriveAsync(photoStreamMS.ToArray());
MessageAttachmentsCollectionPage attachments = new MessageAttachmentsCollectionPage();
attachments.Add(new FileAttachment
{
ODataType = "#microsoft.graph.fileAttachment",
ContentBytes = photoStreamMS.ToArray(),
ContentType = "image/png",
Name = "me.png"
});
// Get the sharing link and insert it into the message body.
Permission sharingLink = await GetSharingLinkAsync(photoFile.Id);
string bodyContentWithSharingLink = String.Format(bodyContent, sharingLink.Link.WebUrl);
// Prepare the recipient list
string[] splitter = { ";" };
var splitRecipientsString = recipients.Split(splitter, StringSplitOptions.RemoveEmptyEntries);
List<Recipient> recipientList = new List<Recipient>();
foreach (string recipient in splitRecipientsString)
{
recipientList.Add(new Recipient { EmailAddress = new EmailAddress { Address = recipient.Trim() } });
}
try
{
var graphClient = AuthenticationHelper.GetAuthenticatedClient();
var email = new Message
{
Body = new ItemBody
{
Content = bodyContentWithSharingLink,
ContentType = BodyType.Html,
},
Subject = subject,
ToRecipients = recipientList,
Attachments = attachments
};
try
{
await graphClient.Me.SendMail(email, true).Request().PostAsync();
}
catch (ServiceException exception)
{
throw new Exception("We could not send the message: " + exception.Error == null ? "No error message returned." : exception.Error.Message);
}
}
catch (Exception e)
{
throw new Exception("We could not send the message: " + e.Message);
}
}
Click on Connect Button from iOS/Android /Windows
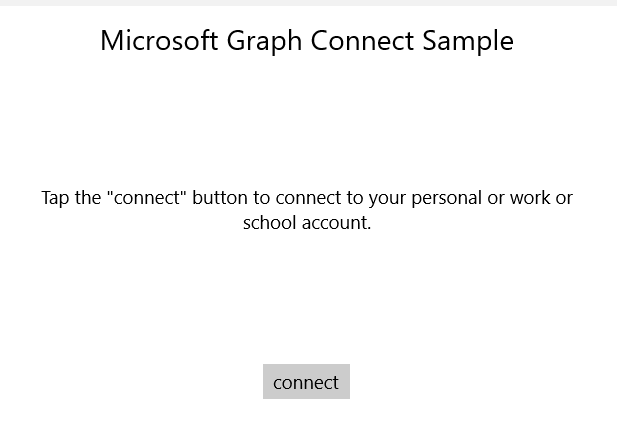
Sign in with your personal or work or school account and grant the requested permissions.

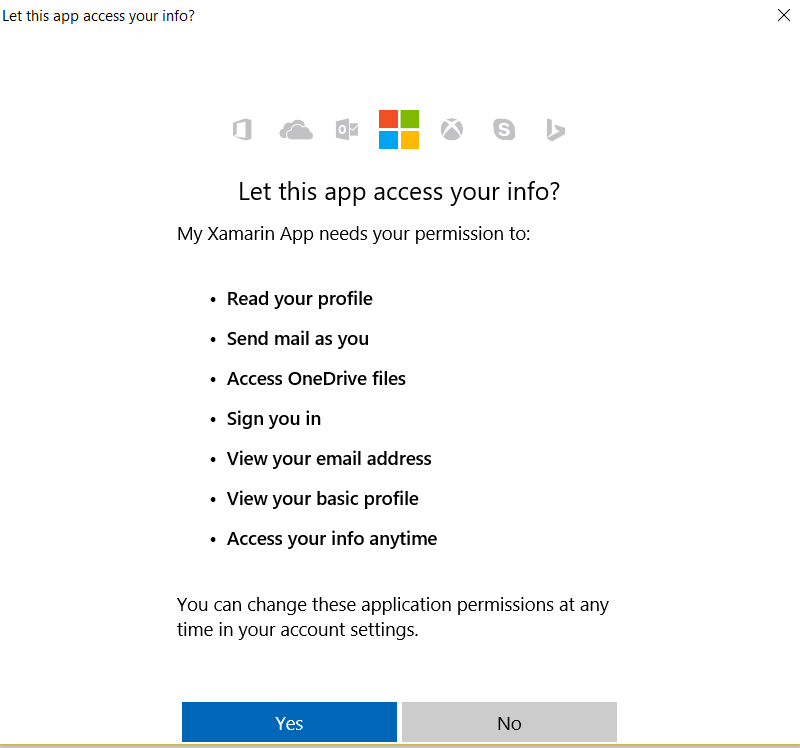
4. Click on Send mail button. When the mail is sent, a Success message is displayed.

This mail message includes the photo as an attachment and also provides a sharing link to the uploaded file in OneDrive. Check your mail from Inbox or Spam.

public async Task ComposeAndSendMailAsync(string subject,string bodyContent,string recipients)
{
// Get current user photo
Stream photoStream = await GetCurrentUserPhotoStreamAsync();
// If the user doesn't have a photo, or if the user account is MSA, we use a default photo
if (photoStream == null)
{
var assembly = typeof(MailHelper).GetTypeInfo().Assembly;
photoStream = assembly.GetManifestResourceStream("XamarinConnect.test.jpg");
}
MemoryStream photoStreamMS = new MemoryStream();
// Copy stream to MemoryStream object so that it can be converted to byte array.
photoStream.CopyTo(photoStreamMS);
DriveItem photoFile = await UploadFileToOneDriveAsync(photoStreamMS.ToArray());
MessageAttachmentsCollectionPage attachments = new MessageAttachmentsCollectionPage();
attachments.Add(new FileAttachment
{
ODataType = "#microsoft.graph.fileAttachment",
ContentBytes = photoStreamMS.ToArray(),
ContentType = "image/png",
Name = "me.png"
});
// Get the sharing link and insert it into the message body.
Permission sharingLink = await GetSharingLinkAsync(photoFile.Id);
string bodyContentWithSharingLink = String.Format(bodyContent, sharingLink.Link.WebUrl);
// Prepare the recipient list
string[] splitter = { ";" };
var splitRecipientsString = recipients.Split(splitter, StringSplitOptions.RemoveEmptyEntries);
List<Recipient> recipientList = new List<Recipient>();
foreach (string recipient in splitRecipientsString)
{
recipientList.Add(new Recipient { EmailAddress = new EmailAddress { Address = recipient.Trim() } });
}
try
{
var graphClient = AuthenticationHelper.GetAuthenticatedClient();
var email = new Message
{
Body = new ItemBody
{
Content = bodyContentWithSharingLink,
ContentType = BodyType.Html,
},
Subject = subject,
ToRecipients = recipientList,
Attachments = attachments
};
try
{
await graphClient.Me.SendMail(email, true).Request().PostAsync();
}
catch (ServiceException exception)
{
throw new Exception("We could not send the message: " + exception.Error == null ? "No error message returned." : exception.Error.Message);
}
}
catch (Exception e)
{
throw new Exception("We could not send the message: " + e.Message);
}
}
Step 9: Run the Application:
Select iOS,android and Windows project and run the application .Click on Connect Button from iOS/Android /Windows
Sign in with your personal or work or school account and grant the requested permissions.
4. Click on Send mail button. When the mail is sent, a Success message is displayed.
This mail message includes the photo as an attachment and also provides a sharing link to the uploaded file in OneDrive. Check your mail from Inbox or Spam.
Msgclub allow you to send 1, 100, 1000000 or unlimited number of SMS with our bulk text SMS plugins.
ReplyDeleteSMS plugins